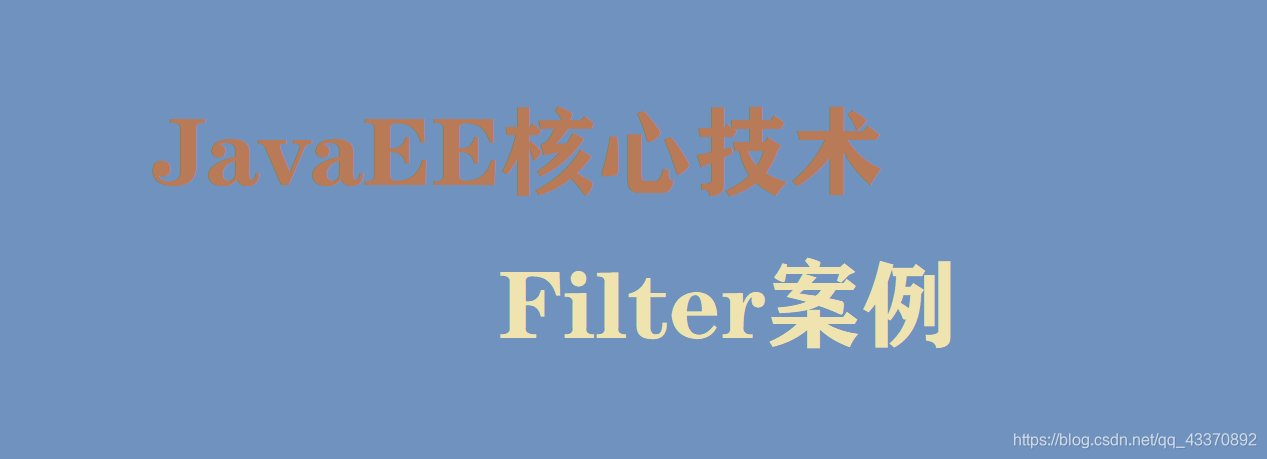
案例一:登录验证
需求
1. 访问项目案例的资源。验证其实是否登录、
2. 如果登录直接放行
3. 如果没有登录,则会跳转到登录页面,提示“您尚未登录,请先登录”。
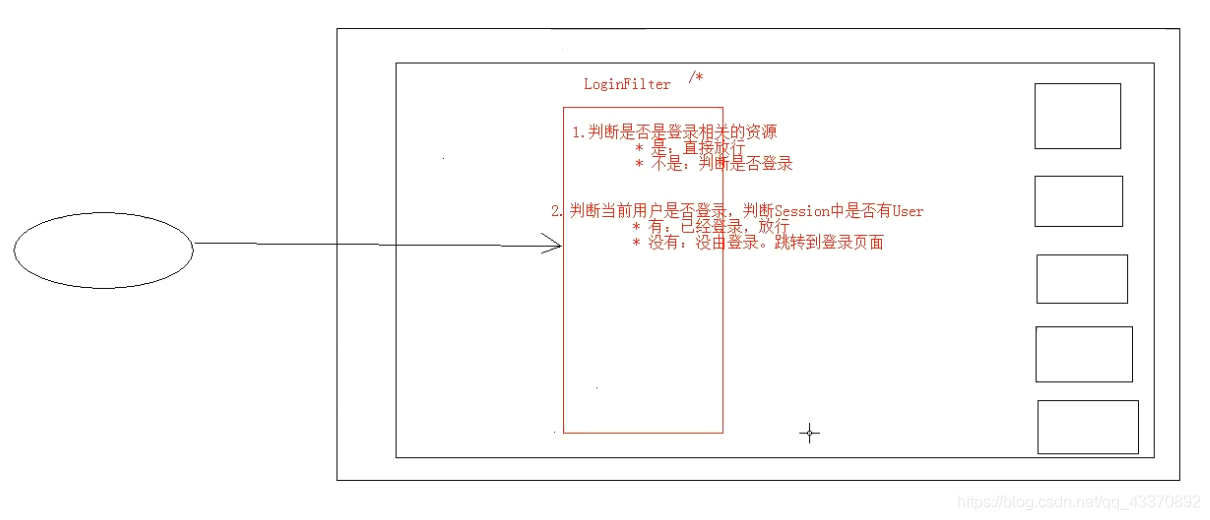
Filter代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| package cn.itcast.web.filter;
import javax.servlet.*; import javax.servlet.annotation.WebFilter; import javax.servlet.http.HttpServletRequest; import java.io.IOException;
@WebFilter(urlPatterns = "/*") public class LoginFilter implements Filter {
@Override public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws ServletException, IOException {
HttpServletRequest request = (HttpServletRequest) req;
String uri = request.getRequestURI();
if (uri.contains("/login.jsp") || uri.contains("/loginServlet") || uri.contains("/css/") || uri.contains("/js/") || uri.contains("/fonts/") || uri.contains("/checkCodeServlet")) { chain.doFilter(req, resp); }else { Object user = request.getSession().getAttribute("user");
if (user != null) { chain.doFilter(req, resp);
}else{ request.setAttribute("login_msg","您尚未登录,请登录!"); System.out.println(request.getAttribute("login_msg")); request.getRequestDispatcher("/login.jsp").forward(request,resp); } } }
@Override public void init(FilterConfig config) throws ServletException {
}
@Override public void destroy() { }
}
|
案例二:敏感词过滤
需求
1. 对案例录入的数据进行敏感词过滤
2. 敏感词参考《敏感词.txt》
3. 如果为敏感词,则替换为 ***
分析
1. 对request对象进行增强。增强获取参数相关参数
2. 放行。传递代理对象。
增强对象的功能
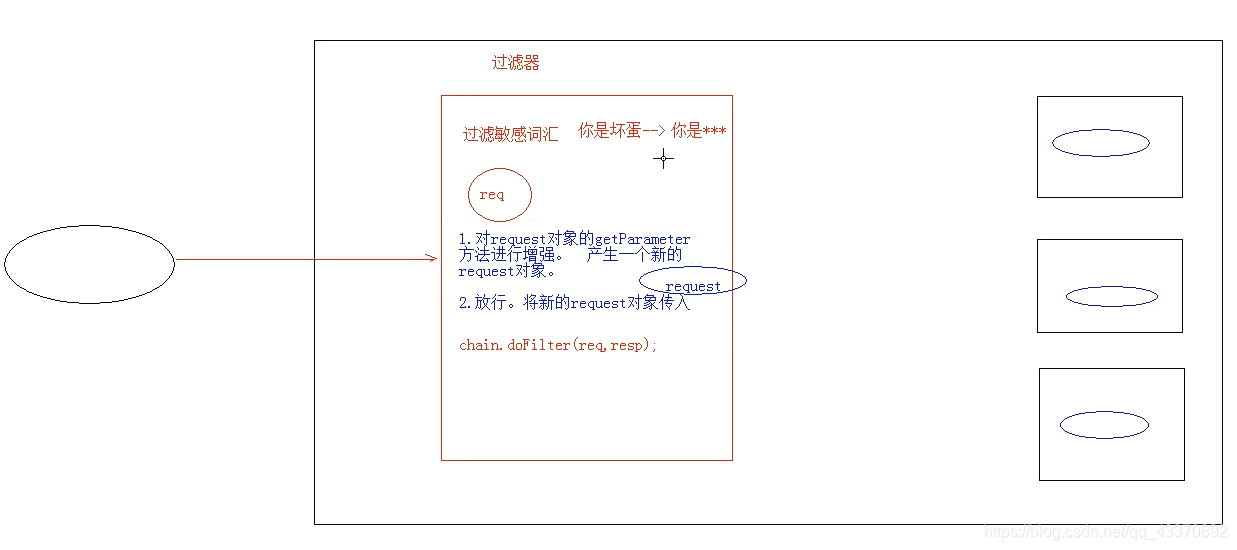
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84
| package com.web.filter;
import javax.servlet.*; import javax.servlet.annotation.WebFilter; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.lang.reflect.InvocationHandler; import java.lang.reflect.Method; import java.lang.reflect.Proxy; import java.util.ArrayList; import java.util.List;
@WebFilter(urlPatterns = "/*") public class SensitiveWordsFilter implements Filter {
@Override public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws ServletException, IOException {
ServletRequest proxy_req = (ServletRequest)Proxy.newProxyInstance(req.getClass().getClassLoader(), req.getClass().getInterfaces(), new InvocationHandler() { @Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { if (method.getName().equals("getParamter")){ String value = (String)method.invoke(req,args); if (value!=null){ for (String str:list) { if (value.contains(str)) { value.replaceAll(str,"****"); } } } return value; }
return method.invoke(req,args); } }); chain.doFilter(proxy_req, resp); } private List<String> list = new ArrayList<String>();
@Override public void init(FilterConfig config) throws ServletException { try { ServletContext servletContext = config.getServletContext(); String realPath = servletContext.getRealPath("/WEB-INF/classes/敏感词汇.txt");
BufferedReader br = new BufferedReader(new FileReader(realPath));
String line = null; while((line =br.readLine())!=null){ list.add(line); }
br.close(); System.out.println(list);
} catch (Exception e) { e.printStackTrace(); }
}
@Override public void destroy() { }
}
|
设计模式
一共23种,一些通用的解决问题的方式。
装饰模式
代理模式
概念
1. 真实对象:被代理的对象 (下图背景北京的代理商)
2. 代理对象:(下图西安卖电脑的)
3. 代理模式:代理对象代理真实对象,达到增强真实功能的目的。
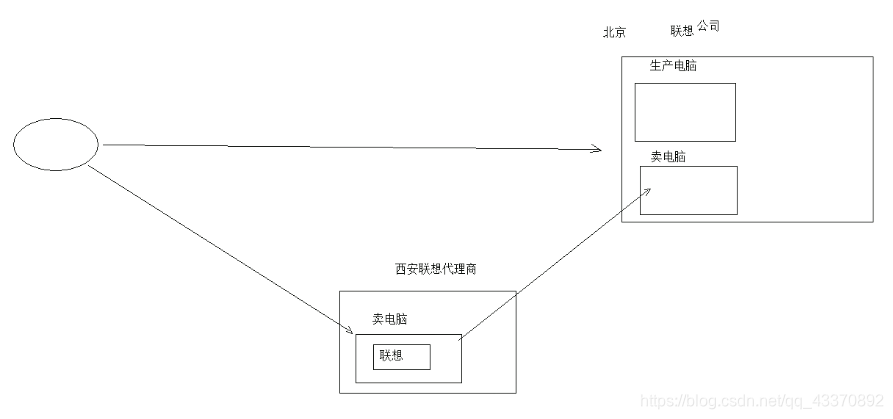
实现方式
1. 静态代理:有一个类文件描述代理模式
2. 动态代理:在内存中来形成代理类
*实现步骤
1. 代理对象和真实对象实现相同的接口
2. 代理对象 = proxy.newProxyInstance();
3. 使用代理对象来调用方法。
4. 增强方法
*增强方式:
1. 增强参数列表
2. 增强返回值类型
3. 增强方法体执行逻辑
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| package cn.itcast.proxy.impl;
import cn.itcast.proxy.SaleComputer;
import java.lang.reflect.*;
public class ProxyTest { public static void main(String[] args) { Lenovo lenovo = new Lenovo();
SaleComputer proxy_lenovo = (SaleComputer) Proxy.newProxyInstance(lenovo.getClass().getClassLoader(), lenovo.getClass().getInterfaces(), new InvocationHandler() {
@Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
if (method.getName().equals("sale")) { double money = (double) args[0]; money = money*0.85;
System.out.println("专车接你。。。"); String obj = (String) method.invoke(lenovo, money); System.out.println("免费送货。。。"); return obj+"_鼠标垫";
}else{
Object obj = method.invoke(lenovo, args); return obj; } } });
String computer = proxy_lenovo.sale(7000); System.out.println(computer); proxy_lenovo.show(); } }
|