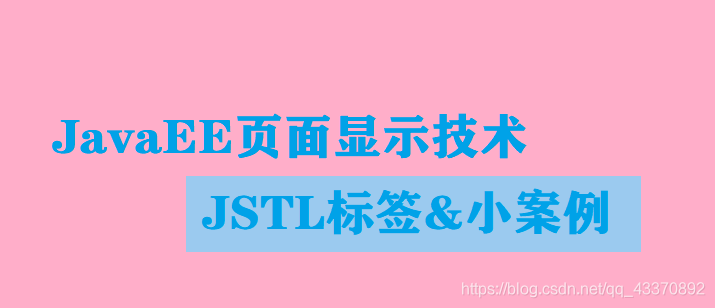
概念
JavaServer Pages Tag Library : JSP标准标签库
* 是由Apache组织提供的开源的免费的JSP标签
作用
用户简化和替换JSP页面上的Java代码
使用步骤
1. 导入JSTL相关的jar包
2. 引入标签库: taglib指令 <%@ taglib ="" prefix=""%>
3. 使用标签
常用的JSTL标签
if
相当于Java代码的if语句
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <%-- 1.test 必须属性,接受boolean表达式 如果表达式为true,则显示if标签体内容,如果为false,则不显示标签体内容。 一般情况下,test属性值会结合el表达式一起使用
2.没有else,若是想用else,则只能再写一份<c:if ...>
--%> <c:if test="true"> 我是真... </c:if>
<% //判断request域中一个list集合为空,如果不为空,则显示遍历集合 List list = new ArrayList(); list.add("aaa"); request.setAttribute("number",3); request.setAttribute("list",list); %>
<c:if test="${not empty list}"> 遍历集合 </c:if>
<c:if test="${number % 2 !=0}"> ${number}为奇数 </c:if>
<c:if test="${number % 2 ==0}"> ${number}为偶数 </c:if>
|
choose
相当于 Java代码的switch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <%-- 完成对应数字编号对应星期几的案例 1. 域中c储存一数字 2. 使用choose标签取出数字, 相当于switch声明 3. 使用when标签做出判断, 相当于case 4. otherwise标签做其他情况的声明 ,相当于default --%> <% request.setAttribute("number",3); %>
<c:choose> <c:when test="number==1">星期一</c:when> <c:when test="number==2">星期二</c:when> <c:when test="number==3">星期三</c:when> <c:when test="number==4">星期四</c:when> <c:when test="number==5">星期五</c:when> <c:when test="number==6">星期六</c:when> <c:when test="number==7">星期天</c:when> <c:otherwise>输入有误</c:otherwise>
</c:choose>
|
foreach
相当于Java代码的for语句
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| <%-- foreach:相当Java的for语句 1. 完成重复的操作 for(int i = 0;i<10;i++){\
} 属性: begin:开始值 end:结束值 var:临时变量 step:步长 varStatus:循环对象 包含俩对象 index:容器中循环的索引,从0开始 count:循环次数,从1开始
2,遍历容器 Lis<User> list; for(User user : list){
}
--%>
<c:forEach begin="1" end="10" var="1" step="1" varStatus="s"> ${i}<br/> </c:forEach>
<hr/>
<% List list = new ArrayList(); list.add("aaa"); list.add("bbb"); list.add("ccc");
request.setAttribute("list",list);
%>
<c:forEach items="${list}" var="str" varStatus="s">
${s.index} ${s.count} ${str}<br/>
</c:forEach>
|
小案例
需求:
在request域中有一个存有User对象的List集合。
需要使用jstl +el 将集合数据展示到JSP页面的表格table中
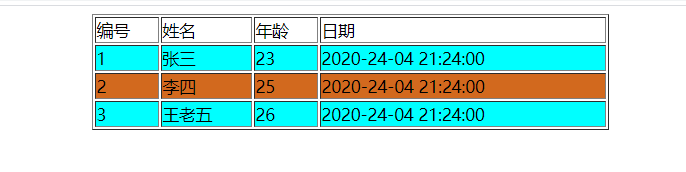
JSP代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| <%@ page import="com.web.domain.User" %> <%@ page import="java.util.ArrayList" %> <%@ page import="java.util.List" %> <%@ page import="java.util.Date" %><%-- Created by IntelliJ IDEA. User: OldAZ-PC Date: 2020/9/4 Time: 21:04 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>案例</title> </head> <body>
<% List list = new ArrayList(); list.add(new User("张三",23,new Date())); list.add(new User("李四",25,new Date())); list.add(new User("王老五",26,new Date())); request.setAttribute("list",list);
%>
<table border="1px" width="500" align="center"> <tr> <td>编号</td> <td>姓名</td> <td>年龄</td> <td>日期</td> </tr> <c:forEach items="${list}" var="user" varStatus="s"> <c:if test="${s.count%2==0}"> <tr bgcolor="#d2691e"> <td>${s.count}</td> <td>${user.name}</td> <td>${user.age}</td> <td>${user.birStr}</td> </c:if>
<c:if test="${s.count%2!=0}"> <tr bgcolor="#00ffff"> <td>${s.count}</td> <td>${user.name}</td> <td>${user.age}</td> <td>${user.birStr}</td> </c:if> </tr> </c:forEach> </table> </body> </html>
|
User代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| package com.web.domain;
import java.text.SimpleDateFormat; import java.util.*;
public class User {
private String name; private int age; private Date birthday;
public User() { }
public User(String name, int age, Date birthday) { this.name = name; this.age = age; this.birthday = birthday; }
public String getBirStr(){ if (birthday != null) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-mm-dd HH:mm:ss"); return sdf.format(birthday); }else{ return ""; } }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public Date getBirthday() { return birthday; }
public void setBirthday(Date birthday) { this.birthday = birthday; }
}
|